Unit Testing in Angular
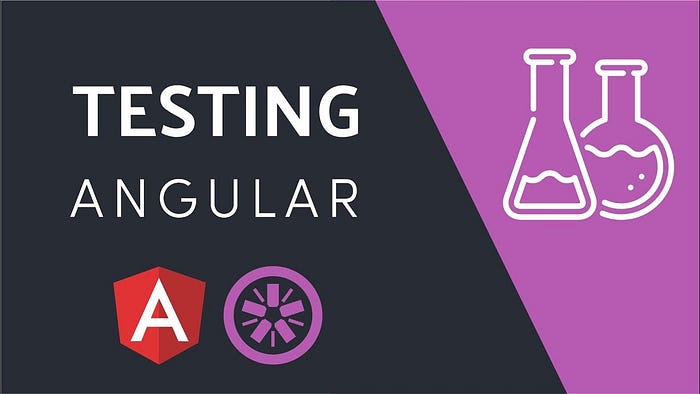
Unit testing is a critical part of the software development process, and it is especially important when working with Angular components. Unit tests help to ensure that your code is working correctly and that it will continue to work correctly even as you make changes or updates to your application.
In this article, we will go over how to write unit tests for Angular components using the Angular Testing Library and the Jasmine test framework.
To get started, you will need to have a basic understanding of Angular components and how they work. If you are new to Angular, you may want to familiarize yourself with the basics before diving into unit testing.
The first step in writing unit tests for Angular components is to set up your testing environment. This involves installing the necessary dependencies, including the Angular Testing Library and the Jasmine test framework. You will also need to configure your testing environment, including setting up the test runner and creating a test file for your components.
Once you have your testing environment set up, you can begin writing your unit tests. To test an Angular component, you will need to create a test bed using the Angular Testing Library. The test bed is responsible for creating a testing environment for your component, including injecting the necessary dependencies and setting up the component’s template.
Once you have your test bed set up, you can start writing your tests. When writing unit tests for Angular components, it is important to focus on testing the component’s behavior rather than its implementation. This means that you should test the component’s inputs and outputs, as well as its interactions with the template and its dependencies.
One way to test an Angular component’s behavior is to use the Angular Testing Library’s “fakeAsync” function. This function allows you to test asynchronous code, such as HTTP requests or setTimeout calls, in a synchronous way.
Here is an example of how you might use the “fakeAsync” function to test an Angular component that makes an HTTP request:
// Filename: mycomponent.component.spec.ts
import { fakeAsync, tick } from '@angular/core/testing';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
let httpClient: HttpClient;
let httpTestingController: HttpTestingController;
beforeEach(fakeAsync(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
declarations: [MyComponent]
}).compileComponents();
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
httpClient = TestBed.inject(HttpClient);
httpTestingController = TestBed.inject(HttpTestingController);
}));
it('should make an HTTP request', fakeAsync(() => {
component.ngOnInit();
tick();
const req = httpTestingController.expectOne('/api/data');
expect(req.request.method).toEqual('GET');
req.flush({ data: 'test data' });
httpTestingController.verify();
}));
});
In this example, we are using the “fakeAsync” function to wrap the setup of our test bed and our test itself. This allows us to use the “tick” function to advance the clock and trigger any asynchronous code that may be running in our component.
We are also using the “HttpTestingController” to mock an HTTP request and verify that it was made with the correct method and URL.
Another way to test an Angular component is to use the “detectChanges” function to trigger a change detection cycle and update the component’s template. This is useful for testing the component’s interactions with the template and for testing the component’s output bindings.
Here is an example of how you might use the “detectChanges” function to test an Angular component’s output binding:
import { ComponentFixture, fakeAsync, TestBed } from '@angular/core/testing';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
beforeEach(fakeAsync(() => {
TestBed.configureTestingModule({
declarations: [MyComponent]
}).compileComponents();
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
}));
it('should update the name output', fakeAsync(() => {
component.name = 'Test Name';
fixture.detectChanges();
expect(fixture.nativeElement.querySelector('#name').textContent).toEqual('Test Name');
}));
});
In this example, we are using the “detectChanges” function to trigger a change detection cycle and update the component’s template. We are then using the nativeElement property of the fixture to query the component’s template and verify that the output binding is correct.
There are many other ways to test Angular components, and the specific tests you write will depend on the needs of your application. However, these are some of the basic techniques that you can use to get started with unit testing your Angular components.
In conclusion, unit testing is an important part of the software development process, and it is essential for ensuring that your Angular components are working correctly. By setting up your testing environment, using the Angular Testing Library and the Jasmine test framework, and focusing on testing the component’s behavior rather than its implementation, you can write effective unit tests for your Angular components.